🧰
prep
Get setup with the main resources you’ll need to learn programming
🧰 Install Node with nvm
Learning Objectives
If you get stuck on any of the below or above instructions, please post in your class channel on Slack.
💡 tip
node -v
in a terminal. The command should return a version number. If it does, you can skip the next steps.🐧 On Ubuntu
- Install nvm by running the following commands in your terminal:
curl -o- https://raw.githubusercontent.com/nvm-sh/nvm/v0.40.1/install.sh | bash
- After the installation is complete, you’ll need to source the nvm script by running:
source ~/.bashrc
- Install the latest LTS version of Node.js by running:
nvm install --lts
- Check that you have successfully installed Node.js by running:
node -v
You should see a version number like v22.11.0
.
- Check that you have successfully installed npm by running:
npm -v
You should see a version number like 10.9.0
.
On Mac
- Install the the Xcode Command Line Developer Tools by running the following command in your terminal:
xcode-select --install
These may already be installed, in which case you will see “xcode-select: note: Command line tools are already installed.” and can continue to the next step.
- Create a (Non-Login Interactive) Shell Configuration File:
touch ~/.zshrc
- Install nvm:
curl -o- https://raw.githubusercontent.com/nvm-sh/nvm/v0.40.1/install.sh | bash
- After the installation is complete, you’ll need to source the nvm script by running:
source ~/.zshrc
- Install the latest LTS version of Node.js by running:
nvm install --lts
- Check that you have successfully installed Node.js by running:
node -v
You should see a version number like v22.11.0
.
- Check that you have successfully installed npm by running:
npm -v
You should see a version number like 10.9.0
.
💡 Protip
Interacting with computers
Learning Objectives
Modern computers are complicated: it would be too difficult and time-consuming to list all the components that make up a modern computer. So to build our mental model, we will use this simple definition of a computer:
A computer is a device used to store and perform operations on data.
🕹️ Using an interface
Learning Objectives
We want to use computers without understanding exactly how they are built. Every day we ask machines to do things, and usually we have no idea how these machines work. We could not use modern technology if we had to understand it completely before we could use it; it would take too long! Instead we use
Think about a cash machine (ATM). We go to a hole in the wall with a screen and a keypad. The screen and the keypad are the user interface. We press the buttons and ask the machine to do things - like giving our balance, or withdrawing some money from an account. We don’t need to understand how the information it tells us comes on the screen.
Exercise
Define the user interface for these devices:
- a calculator
- a microwave
- a desktop lamp
- Alexa
- ChatGPT
🖥️ Terminal interface
Learning Objectives
Programmers need interfaces to ask computers to do things. A computer terminal is an interface where programmers can issue commands to a computer. Because users enter text instructions and receive text output, we say that the terminal is a text-based interface.
Interface via the terminal
We can input a command into the prompt and hit enter. The terminal then passes this command to the computer to execute. Find your own terminal and input the ls
command:
ls
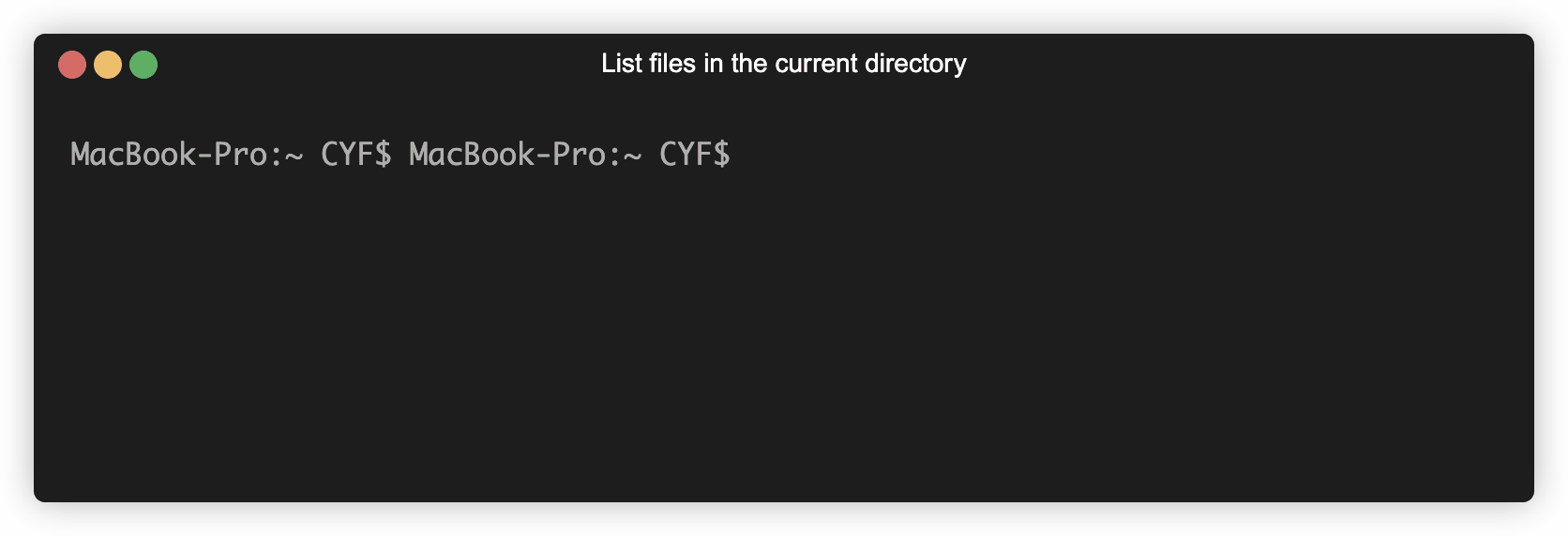
🖊️ Writing computer instructions
We can issue commands to the computer using the terminal. These commands are instructions that the computer knows how to interpret.
The computer knows ls
means “list the files and directories in the current directory”.
During the execution of a computer program, a computer will store and modify
ls
is a shell command. Shell is a programming language we use to interact with the files and folders on our computer. You already know at least two more programming languages. Can you name them?
Definition: programming language
🗄️ Classifying data
Learning Objectives
We’re going to focus on the JavaScript programming language.
A programming language organises data with rules so we understand what we can and cannot do with it. Languages split data up into different categories called
Number data type
10
is an example of the number data type.
3.14
is also part of the number data type; both integers (whole numbers) and non-integers are types of number.
-15
is also part of the number data type. Positive and negative numbers, as well as 0
, are all types of number.
String data type
A string is a sequence of characters demarcated by quotes.
"Code Your Future"
🧮 Creating expressions
Think of the numbers 10
and 32
. We could ask questions about these numbers, like: What is the sum of 10 and 32?
Another way to say this is what do 10 and 32 add up to? In English we can say this in many ways, but in JavaScript we can say this using numbers and an operator. Just like in mathematics, “the sum of 10 and 32” can be written as 10 + 32
:
10 + 32
In JavaScript, +
is an
+
represents the operation “make the sum of the numbers”. It symbolises addition.
The combination of symbols 10 + 32
is an
10 + 32
evaluates to the value 42
.
10
is also an expression. It evaluates to the value 10
.
"Code Your Future"
and "Code Your " + "Future"
are also both expressions - both evaluate to the value "Code Your Future"
.
🧾 Evaluating expressions
Learning Objectives
💡 Tip
Computers work by storing and performing operations on data.
Computer programs are built from many expressions. We must understand how expressions are evaluated to understand how computer programs are executed.
We can take an expression like 36 * 45
and ask what it evaluates to. If we know what the *
operator represents (multiplication) and if we understand the arithmetic rules represented by the operation we can evaluate this expression ourselves.
Happily, computers can evaluate expressions for us.
NodeJS is an application that runs JavaScript programs. In other words, NodeJS can understand and execute programs written in JavaScript. One feature of Node is the REPL.
📝 Note
REPL is a special type of program that stands for:
- Read - Users enter some code that Node will read
- Evaluate - Node will then evaluate this code
- Print - Node will print the result to the terminal
- Loop - Node will loop back to the beginning and prompt users to input some more code
With a REPL we can run pieces of code and look at what happens.
We input JavaScript instructions that are then executed by NodeJS. The REPL replies with, or prints out, the result of this execution.
Type each of the following expressions into the REPL one at a time and then press enter to check the result.
10 + 32
32 / 10
🕹️ Activity
In this activity, you’ll check you’re ready to use the Node REPL on your machine.
- Open the terminal on your computer
- Check you’ve got Node installed on your computer
- Start the Node REPL in your terminal
- Enter the expressions and evaluate them using the Node REPL
If you don’t know how to do any of the steps above, then try searching for an appropriate command online. Searching for things when you’re stuck is super important part of being a developer!
🕹️ Activity
Create your own expressions and enter them into the Node REPL.
🧠 Before you type in the expressions, predict what the REPL output will be. Write your prediction down and compare it to the outcome.
Prep dir
Learning Objectives
📂 Create a working directory for the module
- Fork the coursework module (always linked in every backlog) and open it in VSCode.
- In your VSCode terminal, navigate to the root of your project directory.
- Create a new directory called
prep
to store all the files you’ll be working on for this module.
As you work through the module, you’ll be creating files in this directory to code along with the prep content. You are expected to code along with the prep content.
For simple one liners, use the terminal REPL to run the code. For more complex problems, create files in the prep
directory and write the code there. Make commented notes as you go along explaining why you’re doing what you’re doing. Your future self will thank you.
🔑 The most important thing is to secure your understanding
The prep content is designed to help you understand the concepts you’ll be working with in the module. Don’t just read it, code along with it. Also take notes, draw diagrams, pose your own questions and try to answer them.
To really understand programming, you need to write the code yourself, and do the exercises. You must take active part in your learning to succeed.